Command Templates
A Command Template is a special sort of Command that allows for presets to be created.
The power of Command Templates is that you can use a single script to run presets in situations where the user only needs to change one or more properties.
Note: Command Templates cannot have triggers assigned. To run a Command Template you need to assign a trigger to one of the Command Template's Presets.
Creating a Command Template
Open the script you want to convert to Command Template from the SoundFlow Editor.
For example, let's convert this script, which changes the color of the selected tracks in Pro Tools, into a Command Template.
sf.ui.proTools.colorsSelect(
{
colorTarget:"Tracks",
colorNumber: 10,
colorBrightness: "Medium",
}
)
Click the button "Convert to Template" in the SoundFlow Editor.
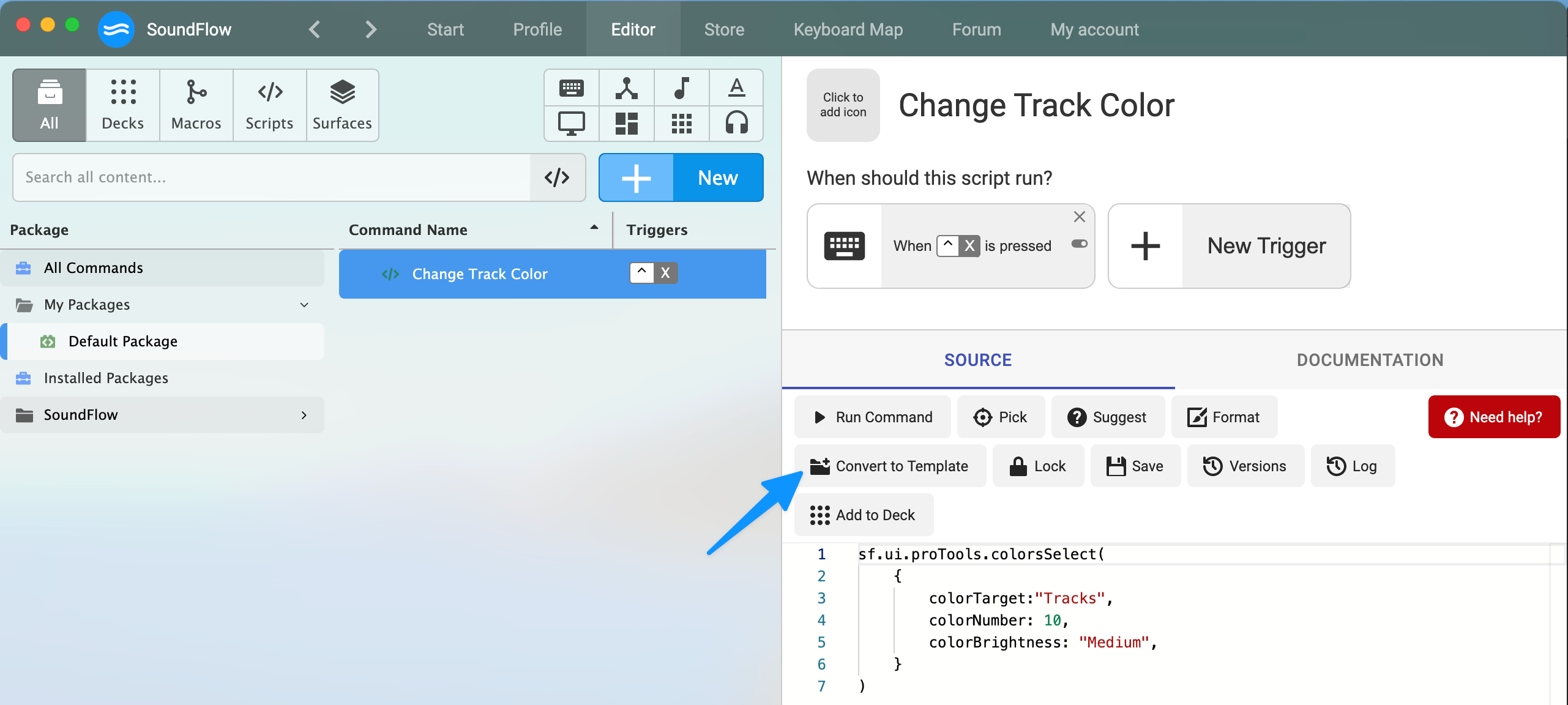
Confirm this choice.
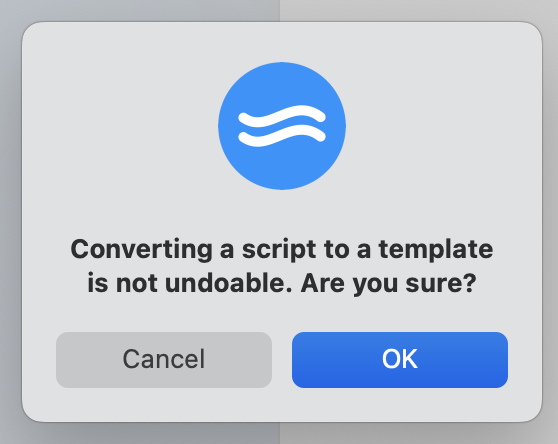
Note: You cannot convert a Command Template into a script. However, you can always copy the source code from the Command Template to a new script in your account if necessary.
Adding Properties
We now have a "Template" option between "Source" and "Documentation" in the Editor. Click this tab to begin adding properties to our Command Template.
Click "Add Property"
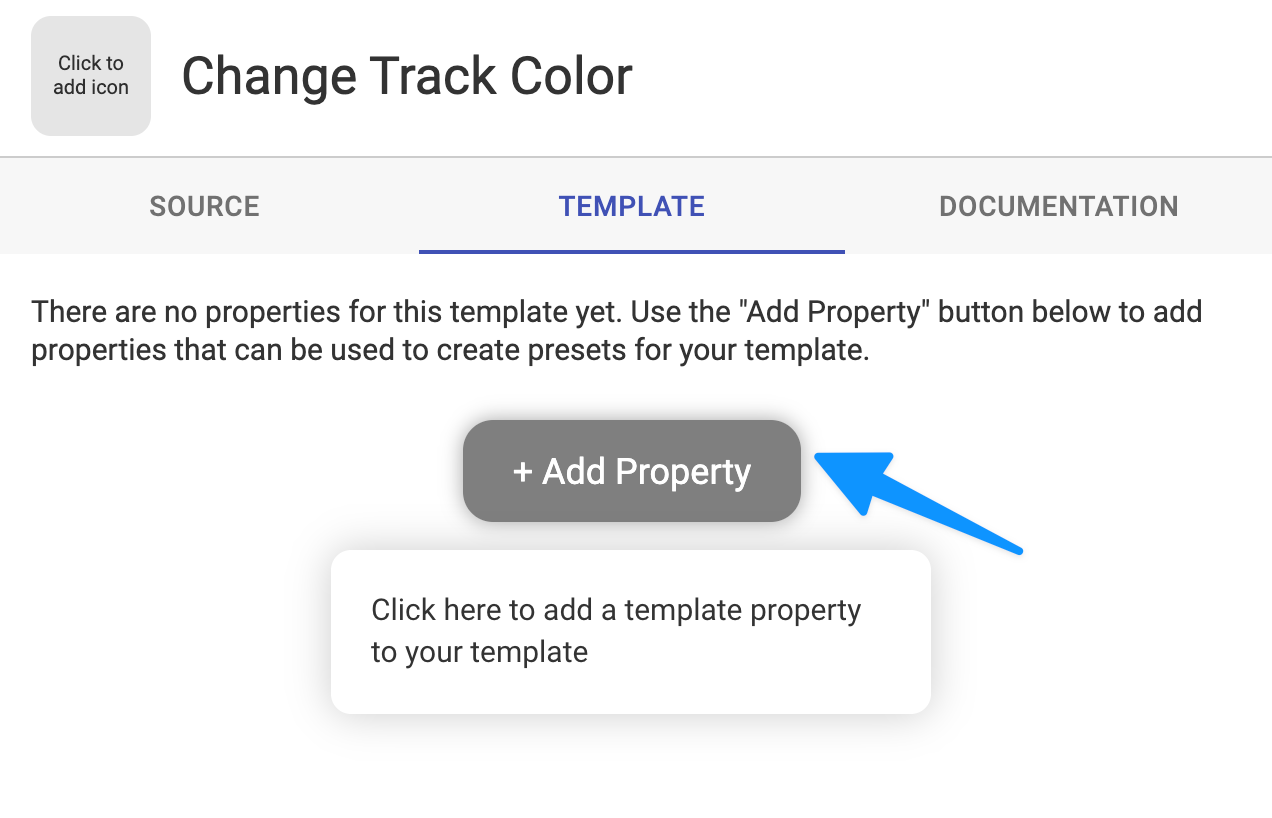
Give this property a title. For this example, we will call it "Track Color Number."
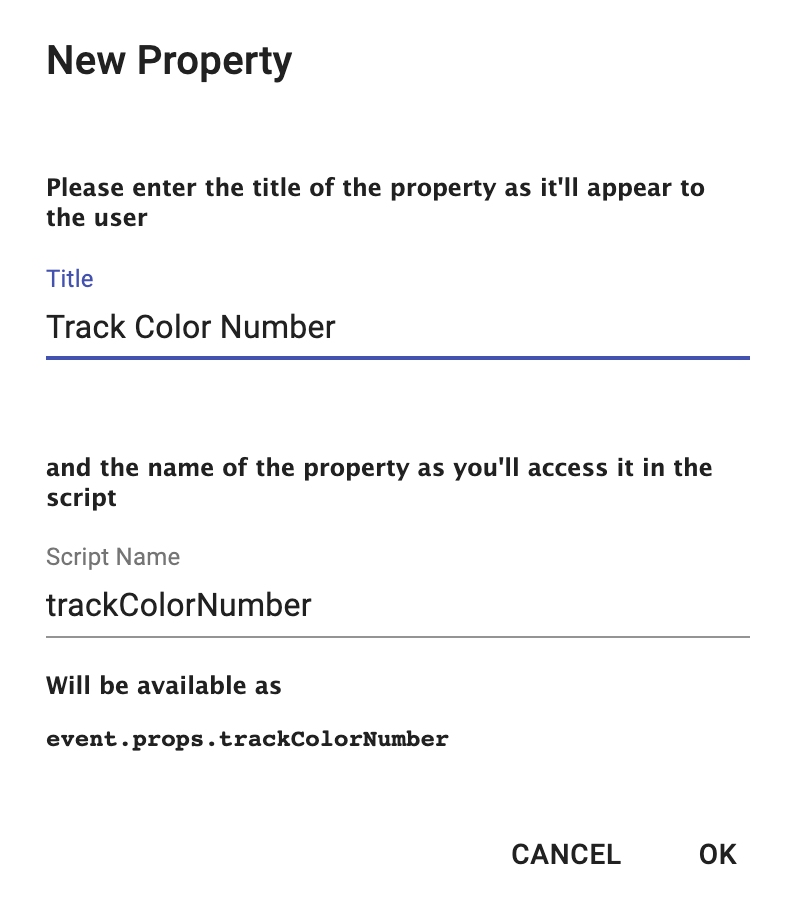
Note: This window also shows the new property will be available via the code event.props.trackColorNumber
in our script.
We can now edit the Type and Default Values for our new Property. Properties can be of type:
AppPath
AxApplication
AxDeck
AxDeckHostDevice
AxDeckOrPreset
AxElement
AxPtAudioSuiteWindow
AxPtTrackHeader
AxPtTrackHeader
AxStreamDeckDevice
AxSurfaceHostDevice
AxSurfaceOrPreset
AxVeProChannel
AxVeProlnstance
AxVeProPlugin
Boolean
Byte
- Callback Function
Double
Float
Int32
Int64
Path
PointF
Short
String
String[]
(String Array)UInt64
- User-Defined Enum
For our purposes, let's configure our Track Color Number
property to have the type Short
(a small number). After selecting the type, we can assign a "Default Value". This can be any value, but for our purposes, we will set this to 10
.
Note: Colors in Pro Tools range from numbers 1
to 23
and can have Light
, Medium
, or Dark
brightness.
We will also create a second property called Track Color Brightness
. For Track Color Brightness
, we will use the type "User-Defined Enum".
The "User-Defined Enum" type allows us to provide multiple options and also define a "Default Value". This allows us to define the options for Light
, Medium
, or Dark
brightness settings.
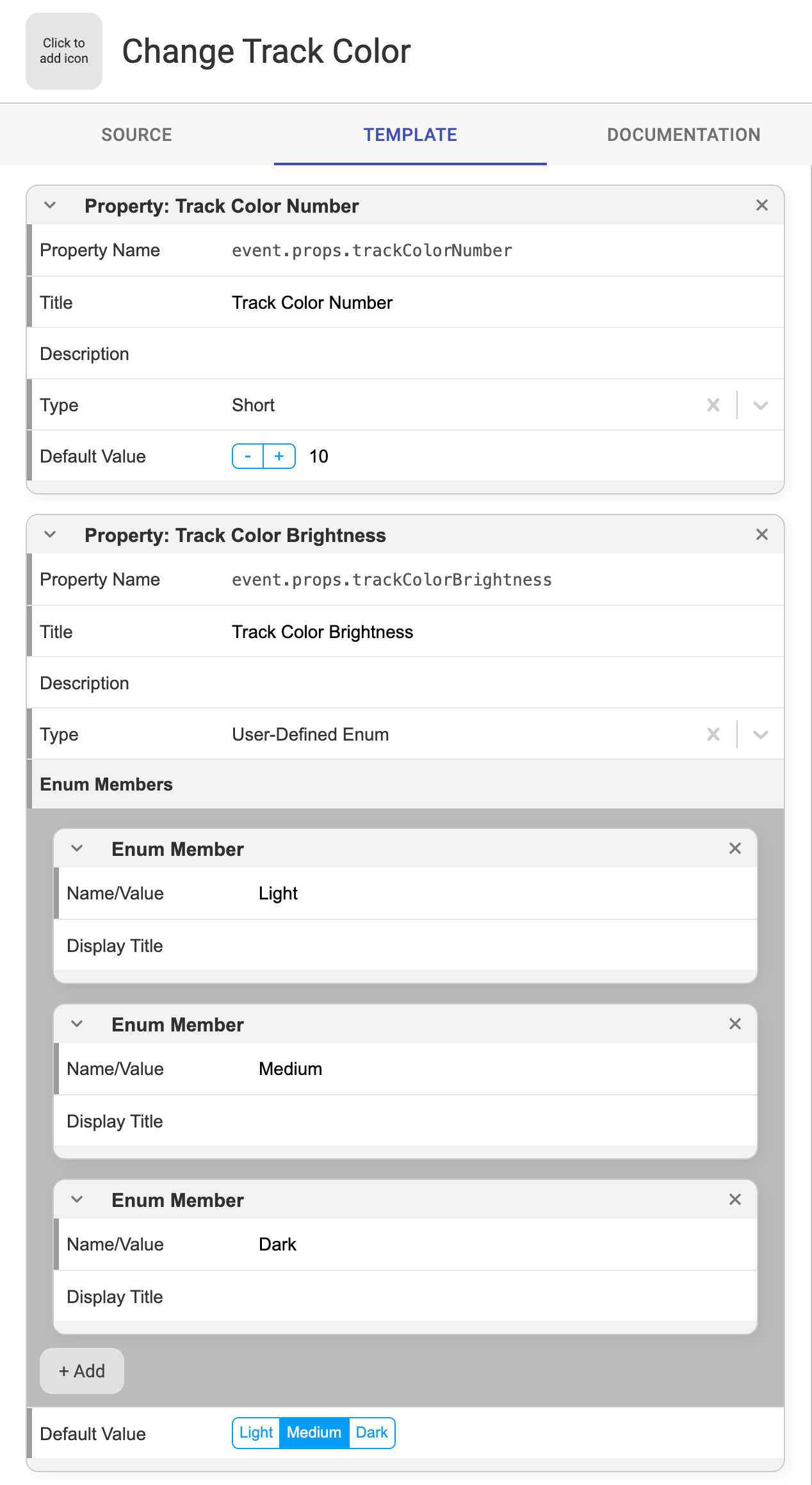
Adding Properties to a Script
To use Command Template properties in a script, we can use Destructuring Assignment. This allows us to define all of our properties in a single line of code like this:
let { trackColorNumber, trackColorBrightness } = event.props;
Once we have defined these properties, we can use them in our script like any other variable. Let's now add the properties to our original script.
So this:
sf.ui.proTools.colorsSelect(
{
colorTarget:"Tracks",
colorNumber: 10,
colorBrightness: "Medium",
}
)
becomes:
let { trackColorNumber, trackColorBrightness } = event.props;
sf.ui.proTools.colorsSelect(
{
colorTarget:"Tracks",
colorNumber: trackColorNumber,
colorBrightness: trackColorBrightness,
}
)
We have replaced the value of the colorNumber
property of the colorSelect()
method with our Template property trackColorNumber
and the value of colorBrightness
property of the colorSelect()
method with our Template property trackColorBrightness
. This allows us to use presets to change these values, increasing the flexibility of our script.
Our Command Template is now ready to use.
Working with Presets
When we converted our script to a Command Template, SoundFlow automatically created a "Factory Presets" folder and a "Default Preset" for our Command Template. The Default Preset will pull it's default values, if set, from the values configured when creating the Template properties.
To add a preset, we can click the "New" button and select "Preset".
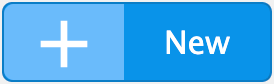
We also have the option to create "Preset Folders" within either the "Factory Presets" folder or the "My Presets" folder.
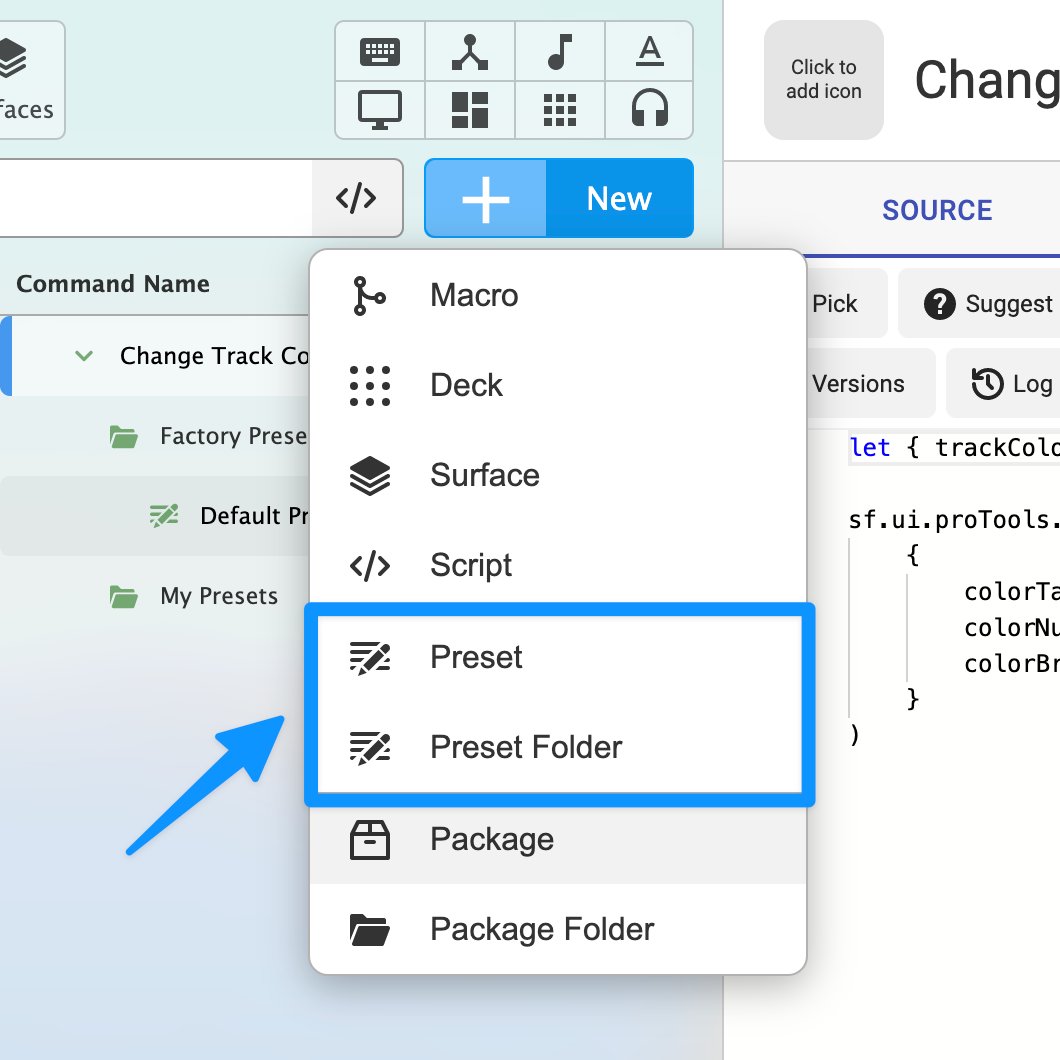
The "Factory Presets" and "My Presets" folders are helpful organizational tools when publishing your Command Templates on the Store.
"Factory Presets" are available to all users of the published Command Template.
"My Presets" are private to your account and can be used to create customized versions of the Command Template.
Presets can be organized further into subfolders allowing for even more flexibility.
Using Command Templates from the Store
There are many packages on the SoundFlow Store utilizing Command Templates allowing you to customize these commands to the needs of your work.
A great example is Teezio's Plugin Loader.
With this app, you can design custom decks to recall your favorite plug-ins and even using the template properties to recall specific plug-in presets.
To create your own presets for this package, select the command in your command list and then click "Add New Preset"
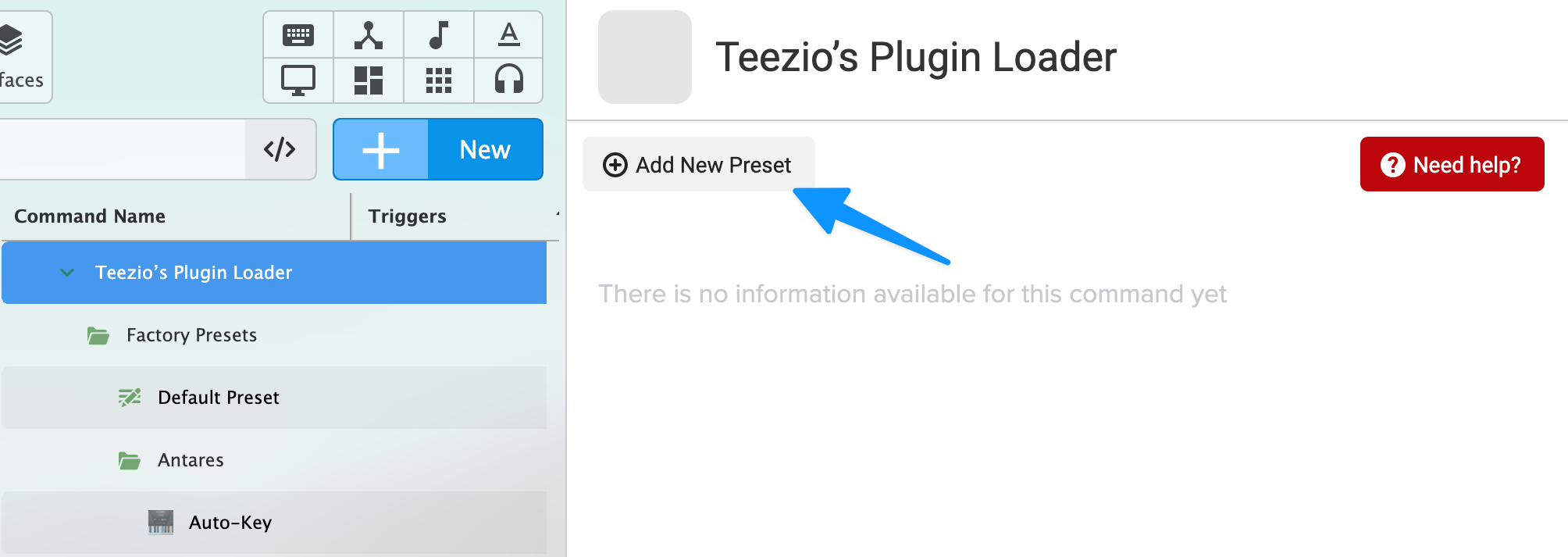
Then, you can fill in the template properties to suit your needs.
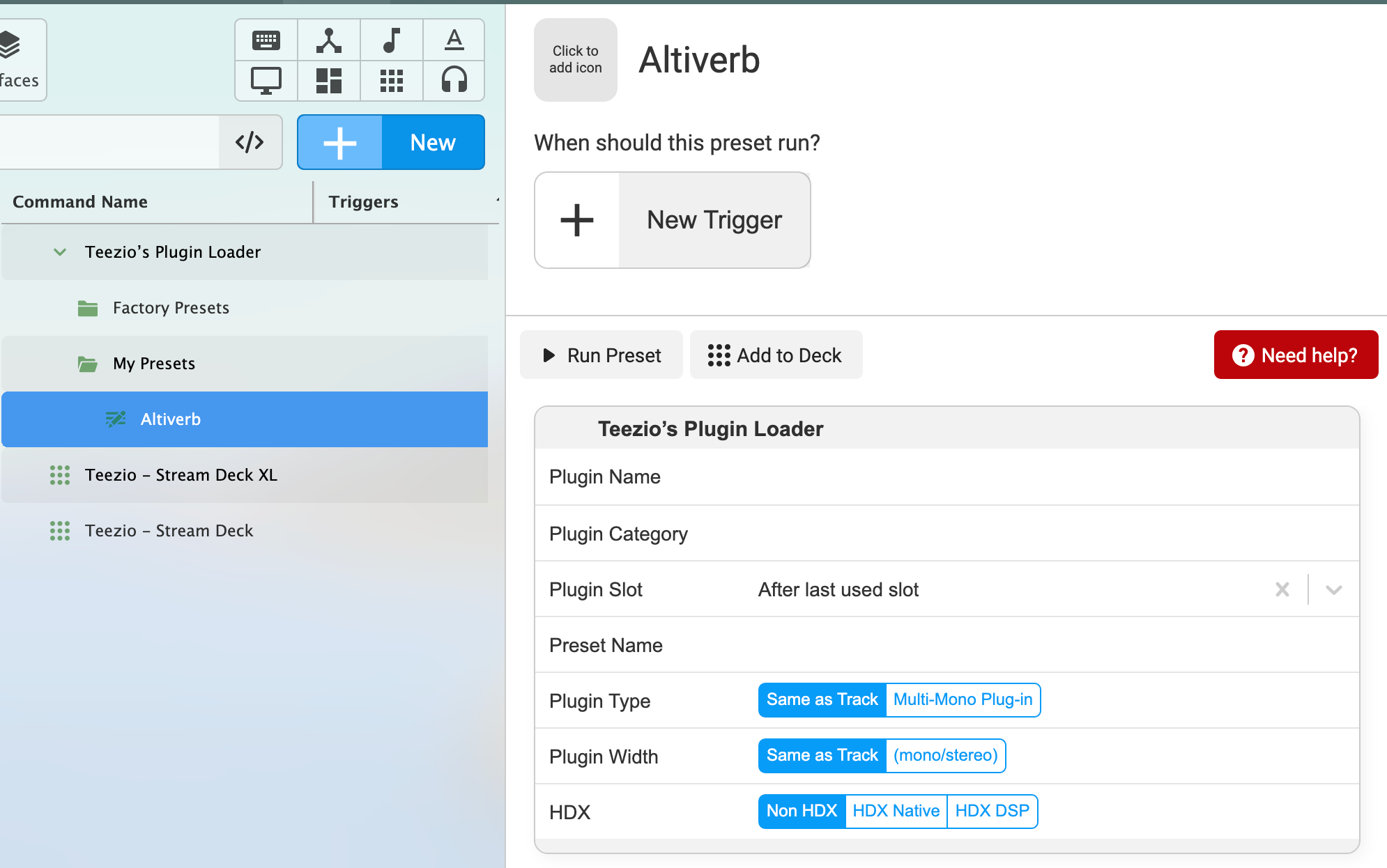
Note: you can also use "Click to Add Icon" to further customize each preset with custom images.
Make Editable Copy of a Command Template
Open-source Command Templates can be copied to other packages by selecting the Command Template in the Commands list and clicking "Make Editable Copy."
This will copy all factory and user presets to a user package allowing for further customization or sharing user presets on the SoundFlow Store.
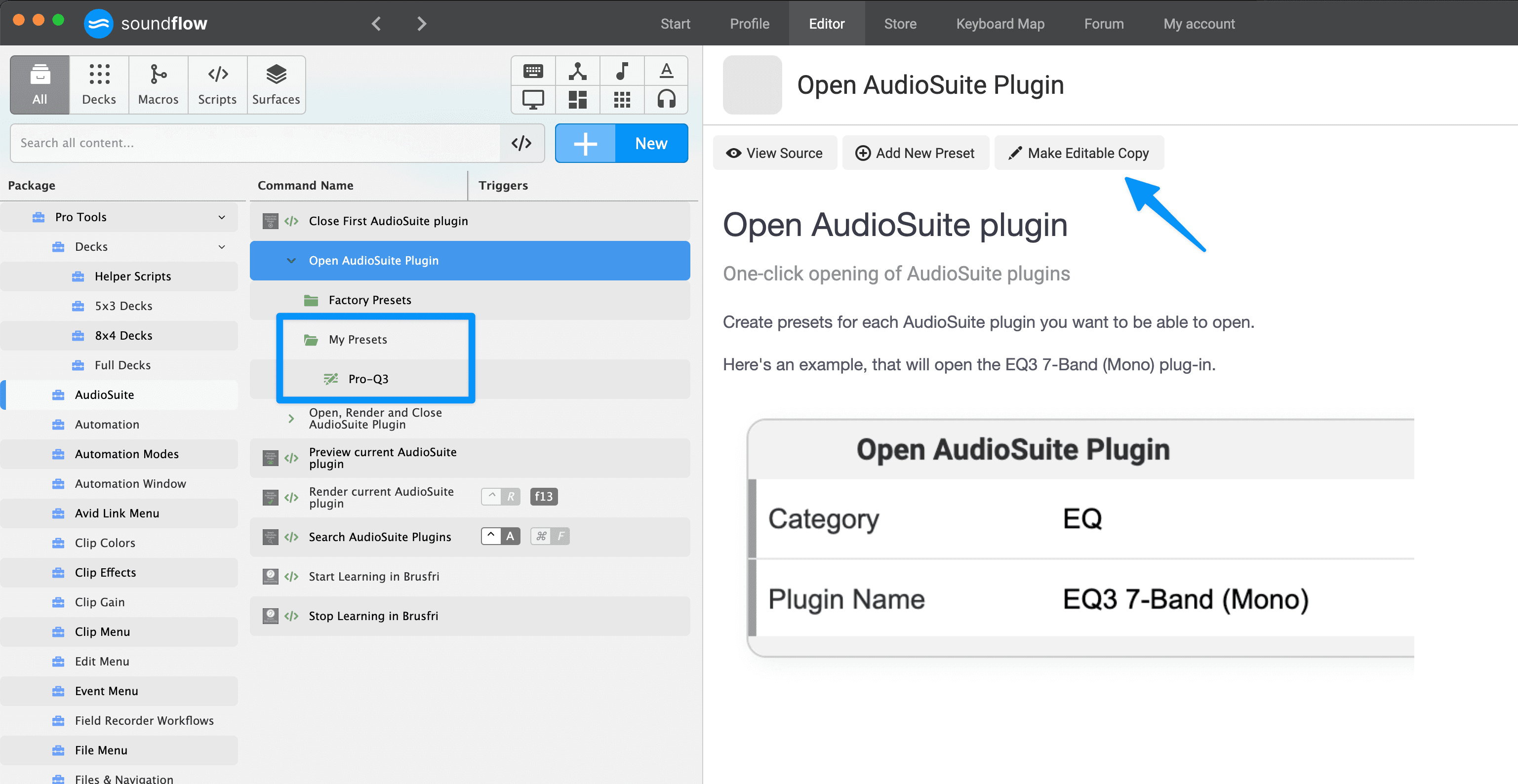